Appearance
Here's the complete Google Apps Script code implementing the requested functions:
Explanation of Functions
settingBMS
:- Centralized settings for parameters like API URL, sheet name, senderId, authToken, delay, etc.
getDataBMS
:- Fetches rows with blank values in the update response and full response columns.
- Limits the fetched rows to
processMessageLimit
.
sendMessageBMS
:- Iterates through rows fetched by
getDataBMS
. - Sends a message using the cURL API with parameters and adds a delay between each message.
- Calls
updateBMS
to log the response.
- Iterates through rows fetched by
updateBMS
:- Updates the Google Sheet with the API response status (
true
orfalse
) and the full response as JSON.
- Updates the Google Sheet with the API response status (
bash
function settingBMS() {
return {
apiBMSurl: 'https://zone-b.botmastersender.com/api/v5/?action=send',
sheetName: 'Sheet1',
messageDelay: 5000, // in milliseconds
senderId: '91700078xxxxx',
authToken: '703-eEfhNBzb9lmkV2sajiREAArV3xxxxxxxxxxxxxxxxxx',
receiverIdCol: 1, // Column number for receiverId
messageTextCol: 2, // Column number for messageText
processMessageLimit: 10, // Number of messages to process
responseCol: 3, // Column number for update response (true/false)
fullResponseCol: 4 // Column number for full API response
};
}
function logProcess(message) {
console.log(`[${new Date().toISOString()}] ${message}`);
}
function getDataBMS() {
const settings = settingBMS();
const sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName(settings.sheetName);
const data = sheet.getDataRange().getValues();
const rowsToProcess = [];
logProcess('Fetching rows to process from the sheet.');
for (let i = 1; i < data.length; i++) {
const responseCol = data[i][settings.responseCol - 1];
const fullResponseCol = data[i][settings.fullResponseCol - 1];
if (!responseCol && !fullResponseCol) {
rowsToProcess.push(i + 1); // Storing row numbers
if (rowsToProcess.length >= settings.processMessageLimit) break;
}
}
logProcess(`Fetched ${rowsToProcess.length} rows to process.`);
return rowsToProcess;
}
function sendMessageBMS() {
const settings = settingBMS();
const sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName(settings.sheetName);
const rowsToProcess = getDataBMS();
rowsToProcess.forEach((row) => {
const receiverId = sheet.getRange(row, settings.receiverIdCol).getValue();
const messageText = sheet.getRange(row, settings.messageTextCol).getValue();
const payload = {
senderId: settings.senderId,
authToken: settings.authToken,
messageText: messageText,
receiverId: receiverId
};
logProcess(`Sending message for row ${row}: receiverId=${receiverId}, messageText=${messageText}`);
const options = {
method: 'post',
contentType: 'application/json',
payload: JSON.stringify(payload)
};
try {
const response = UrlFetchApp.fetch(settings.apiBMSurl, options);
const responseData = JSON.parse(response.getContentText());
logProcess(`Message sent successfully for row ${row}. Response: ${response.getContentText()}`);
updateBMS(row, responseData);
} catch (e) {
console.error(`Error sending message for row ${row}:`, e);
updateBMS(row, { success: false, message: e.message });
logProcess(`Error sending message for row ${row}: ${e.message}`);
}
logProcess(`Waiting for ${settings.messageDelay / 1000} seconds before processing the next message.`);
Utilities.sleep(settings.messageDelay);
});
}
function updateBMS(row, response) {
const settings = settingBMS();
const sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName(settings.sheetName);
// Parse the response correctly
let success = false;
let fullResponse = '';
try {
if (Array.isArray(response)) {
success = response[0]?.success === true;
fullResponse = JSON.stringify(response[0]);
} else {
success = response?.success === true;
fullResponse = JSON.stringify(response);
}
} catch (error) {
logProcess(`Error parsing response for row ${row}: ${error.message}`);
}
logProcess(`Updating sheet for row ${row}. Success: ${success}, Full Response: ${fullResponse}`);
try {
sheet.getRange(row, settings.responseCol).setValue(success ? 'TRUE' : 'FALSE');
sheet.getRange(row, settings.fullResponseCol).setValue(fullResponse);
logProcess(`Row ${row} updated successfully. Success: ${success}`);
} catch (e) {
logProcess(`Error updating sheet for row ${row}: ${e.message}`);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
Setup Instructions
- Copy and paste the code into the Google Apps Script editor.
- Adjust the column numbers and settings in
settingBMS
to match your sheet structure. - Run
sendMessageBMS
to process and send messages in batches.
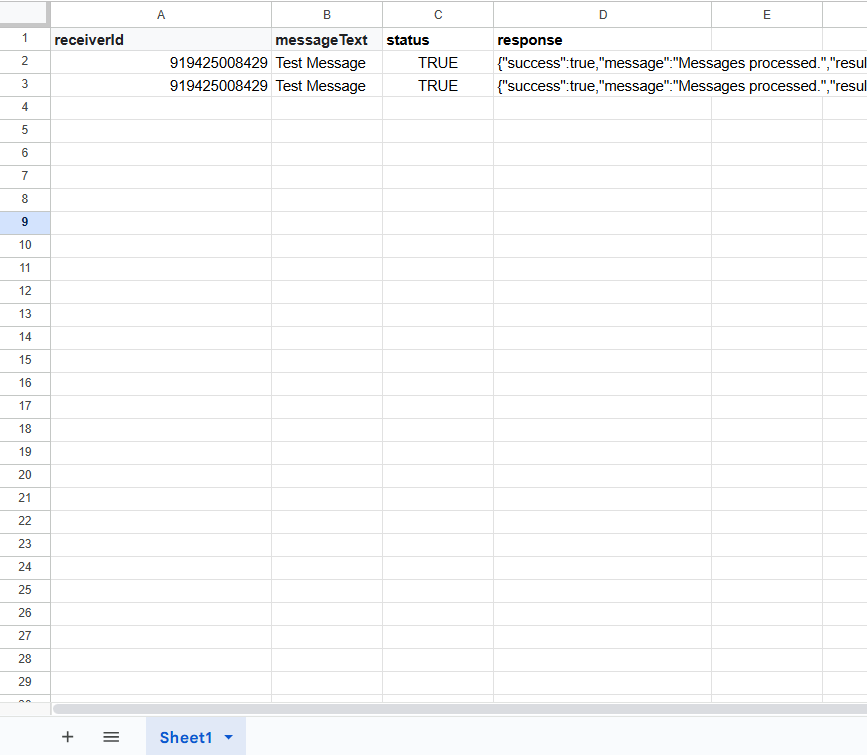